In this quick article, we will discuss step by step how to use Apache HttpClient 4.5 to make an HTTP POST request. The HTTP POST request method requests that the server accepts the entity enclosed in the request as a new subordinate of the web resource identified by the URI. Once you prepared a request, click the Send Request link above the request (this will appear if the file's language mode is HTTP, by default.http files are like this), or use shortcut Ctrl+Alt+R(Cmd+Alt+R for macOS), or right-click in the editor and then select Send Request in the menu, or press F1 and then select/type Rest Client: Send Request, the response will be previewed in a separate. Java REST client example 1 This first example shows a combination of these Apache HttpClient classes used to get information from the Yahoo Weather API. That service actually returns information in an RSS format, but if you don't mind parsing that XML, it's an easy way to get weather updates. Apache HttpClient is a robust and complete solution Java library to perform HTTP operations, including RESTful service. In this tutorial, we show you how to create a RESTful Java client with Apache HttpClient, to perform a “ GET ” and “ POST ” request.
- Apache Rest Client Maven
- Apache Cxf Rest Client
- Apache Rest Client
- Apache Wink Rest Client Example
- Apache Cxf Rest Client Example Mkyong
- Apache Cxf Rest Client Example
- Apache HttpClient Tutorial
- Apache HttpClient Resources
- Selected Reading
Using Secure Socket Layer, you can establish a secured connection between the client andserver. It helps to safeguard sensitive information such as credit card numbers, usernames, passwords, pins, etc.
You can make connections more secure by creating your own SSL context using the HttpClient library.
Follow the steps given below to customize SSLContext using HttpClient library −
Step 1 - Create SSLContextBuilder object
SSLContextBuilder is the builder for the SSLContext objects. Create its object using the custom() method of the SSLContexts class.
Step 2 - Load the Keystore
In the path Java_home_directory/jre/lib/security/, you can find a file named cacerts. Save this as your key store file (with extension .jks). Load the keystore file and, its password (which is changeit by default) using the loadTrustMaterial() method of the SSLContextBuilder class.
Step 3 - build an SSLContext object
An SSLContext object represents a secure socket protocol implementation. Build an SSLContext using the build() method.
Step 4 - Creating SSLConnectionSocketFactory object
SSLConnectionSocketFactory is a layered socket factory for TSL and SSL connections. Using this, you can verify the Https server using a list of trusted certificates and authenticate the given Https server.
You can create this in many ways. Depending on the way you create an SSLConnectionSocketFactory object, you can allow all hosts, allow only self-signedcertificates, allow only particular protocols, etc.
To allow only particular protocols, create SSLConnectionSocketFactory object by passing an SSLContext object, string array representing the protocols need to be supported, string array representing the cipher suits need to be supported and a HostnameVerifier object to its constructor.
To allow all hosts, create SSLConnectionSocketFactory object by passing a SSLContext object and a NoopHostnameVerifier object.
Step 5 - Create an HttpClientBuilder object
Create an HttpClientBuilder object using the custom() method of the HttpClients class.
Step 6 - Set the SSLConnectionSocketFactory object
Set the SSLConnectionSocketFactory object to the HttpClientBuilder using the setSSLSocketFactory() method.
Step 7 - Build the CloseableHttpClient object
Build the CloseableHttpClient object by calling the build() method.
Step 8 - Create an HttpGet object
The HttpGet class represents the HTTP GET request which retrieves the information ofthe given server using a URI.
Create a HTTP GET request by instantiating the HttpGet class by passing a string representing the URI.
Step 9 - Execute the request
Execute the request using the execute() method.
Example
Following example demonstrates the customization of the SSLContrext −
Output
On executing, the above program generates the following output.
To automate the interaction with ACE, you can use its REST client API. Typical use cases include the tight integration of ACE into your development or automated build process, or the creation of a custom user interface or integration.
This is still a work in progress which you can track in ACE-151.
Before we dive into the various REST endpoints, let's start with an overview of how all of this works. Everything in ACE is an entity. For example, an artifact or a feature is an entity, but also the association between an artifact and a feature is. Entities have properties and tags. Properties are the 'fixed' attributes that make up a specific type of entity. For example a feature has a name and a description. If you know the type of entity, its properties are also known. Tags on the other hand are attributes that a user can freely add and refer to.
The following diagram shows the different entities that ACE knows:
For each entity we will now list and explain the properties:
Apache Rest Client Maven
Artifacts contain the metadata for the different types of artifacts that can be provisioned to targets. Artifacts can be bundles, configuration data or any other type you've defined yourself.
- artifactName
- artifactDescription
- url
- mimetype
- processorPid
Since there are different types of artifacts, some properties depend on the actual type.
For bundles:
- Bundle-Name
- Bundle-SymbolicName
- Bundle-Version
For configuration:
- filename
For other types, please consult the documentation about these types (specifically the classes that implement the interfaces in the org.apache.ace.client.repository.helper package).
Associates artifacts to features. Associations have a left and right hand side. Both can be expressed as filter conditions, and both have a cardinality.
- leftEndpoint
- rightEndpoint
- leftCardinality — defaults to 1
- rightCardinality — defaults to 1
A feature is a grouping mechanism for artifacts. It is commonly used to group together a set of artifacts that together represent some kind of feature to the application.
Apache Cxf Rest Client
- name
- description
Associates features to distributions. Associations have a left and right hand side. Both can be expressed as filter conditions, and both have a cardinality.
- leftEndpoint
- rightEndpoint
- leftCardinality — defaults to 1
- rightCardinality — defaults to 1
A feature is a grouping mechanism for features. It is commonly used to group together a set of features that together represent some kind of distribution. Distributions are the things you can associate with targets, so you can look at them as the licensable configurations of your software.
- name
- description
Associates distributions to targets. Associations have a left and right hand side. Both can be expressed as filter conditions, and both have a cardinality.
- leftEndpoint
- rightEndpoint
- leftCardinality — defaults to 1
- rightCardinality — defaults to 1
A target receives the artifacts you provision to it. Most of the time, a target is an OSGi container and provisioning means you'll actually install the artifacts in the container, but there can be other types of targets (non-OSGi containers, or something completely different).
- id
- autoapprove
A target also has specific state (see below for how that is returned):
- registrationState — Unregistered, Registered
- provisioningState — Idle, InProgress, OK, Failed
- storeState — New, Unapproved, Approved
- currentVersion
- isRegistered
- needsApproval
- autoApprove
- artifactsFromShop
- artifactsFromDeployment
- lastInstallSuccess
Similar to the web based UI, this API also works with the concept of checking out a copy to a local working area, altering it in that working area and then committing it back to the server.
POST /work
Creates a working copy for you, with its own ID, and redirects you to it. (For the contents of this working copy, see below.)
Response:
- 302 /work/ID
DELETE /work/ID
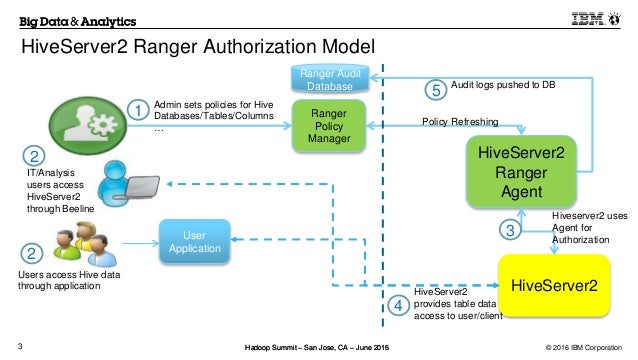
Deletes the working copy. Signals you're done with it, without transferring the data back to the server.
POST /work/ID
Sets the working copy to be the current version. We keep track of whether you have based your copy on, and will reject the update if it is no longer the latest.
Response:
Apache Rest Client
- 200
- 409

Now you have a working copy, you can start working with it.
GET /work/ID
Gets you a list of all repositories you can use. Should return: ['artifact', 'feature', 'distribution', 'target', 'artifact2feature', 'feature2distribution', 'distribution2target']
GET /work/ID/feature
Lists all feature IDs in the feature repository.
POST /work/ID/feature
Creates a new feature. In the post body, you can specify attributes and tags, for example: {attributes: {key: 'value'}, tags: {key: 'value'}}.
Response:
- 302 /work/ID/feature/FID
- 400
GET /work/ID/feature/FID
Returns a representation of the object, for example: {attributes: {key: 'value'}, tags: {key: 'value'}}. If you ask for a target, you will also get its state, so the result will be in the form: {attributes: {key: 'value'}, tags: {key: 'value'}, state: {key: 'value'}}.
PUT /work/ID/feature/FID
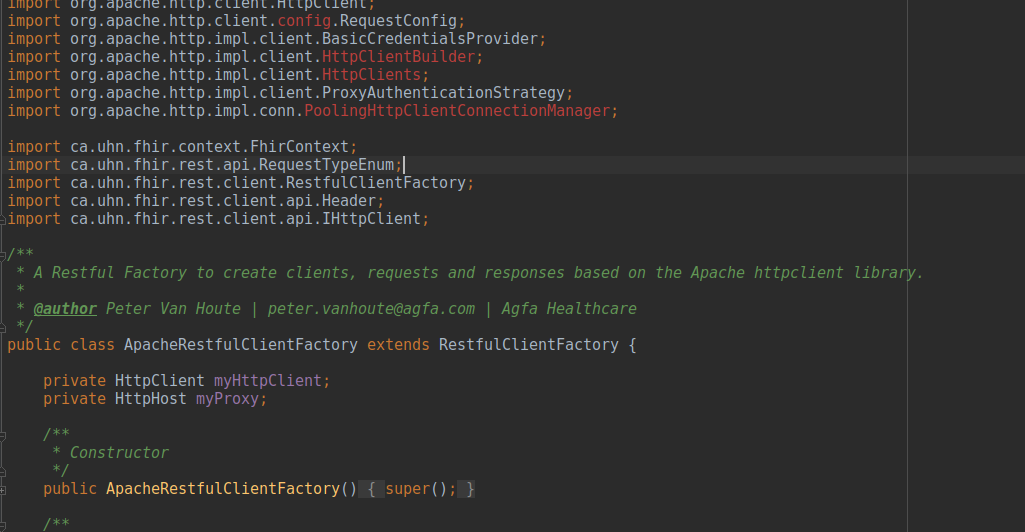
Apache Wink Rest Client Example
Updates the object with the JSON data you supply.
Response:
Apache Cxf Rest Client Example Mkyong
- 200
- 400
- 404
DELETE /work/ID/feature/FID
Deletes the object.
Response:
Apache Cxf Rest Client Example
- 200
- 404
